( 0 ) Download and Install GLUT &
FreeImage for Windows
|
You must first download the GLUT for Windows library.
The file can be downloaded at the following location: GLUT for Win32
The installation instructions for the library are out of date so here
are the proper locations for the files under Visual Studio .NET.
FreeImage can be downloaded for Windows at : Free Image for
Win32
Install Glut into the
following directories:
glut32.dll -> C:\Windows\System or
C:\WinNT\System
glut32.lib -> C:\Program Files\Microsoft Visual
Studio 8\VC\PlatformSDK\lib
glut32.h -> C:\Program Files\Microsoft Visual
Studio 8\VC\PlatformSDK\include\gl
Unpack FreeImage. You
will see a directory called Dist (distribution). These files
should be installed into the following locations. (note the different location for
freeImage.h)
freeImage.dll -> C:\Windows\System or
C:\WinNT\System
freeImage.lib -> C:\Program Files\Microsoft
Visual Studio 8\VC\PlatformSDK\lib
freeImage.h -> C:\Program Files\Microsoft
Visual Studio 8\VC\PlatformSDK\include
Note:
In order for someone else on a different machine to run your
application you must include the glut32.dll file with the
program. If they do not have this file in the same directory as your
application or in thier C:\Windows\System folder and error
will occur and the program will not run.
|
( 1 ) Open a New Project
|
First open Microsoft Visual Studio .NET if you have not
already done so.
Once it is open click File -> New -> Project...
You should now see a dialog box that looks like this:
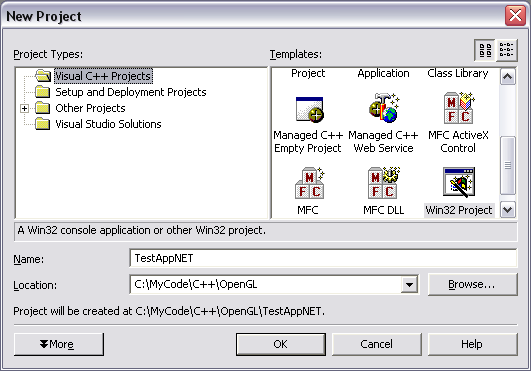
|
( 2 ) Create a New Win32 Project
|
On the left side under Project Types click: Visual
C++ Projects
On the right side under Templates scroll down until you see
the Win32 Project icon. Single click on the icon to
select it.
Enter a name for the project in the Name field below.
Note:
This name will be both the project name and the directory in which to
create the project. (See Project will be created at ... label
at the bottom of the dialog).
Using the Browse... button you can select the directory in
which to put the project.
Once you are satisfied with the settings click: OK
The current dialog will close and the Win32 Application Wizard
will popup.
|
( 3 ) Create a New Console Application
|
In order to create an empty console application
(standard for using OpenGL/GLUT) you need to click on Application
Settings on the left side of the dialog.
The right side of the dialog should now look different. Under the Application
Type heading click Console Application. Under
the Additional Options heading click the Empty
Project checkbox (so it contains a checkmark).
Your dialog should now look similar to the one below. Click Finish.
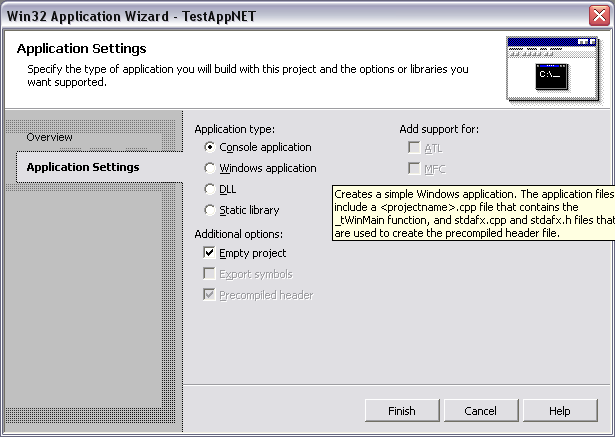
|
( 4 ) Linking OpenGL and GLUT
|
You should now be facing a blank project. Somewhere
within Visual Studio should be the Solution Explorer (It is
docked on the right side at the top by default).
Note:
A Solution is the same as a Workspace if you are
familar with the Visual Studio 6.0/5.0 terminology.
If this windows does not exist click View -> Solution
Explorer or press Ctrl + Alt + L.
It will look similar to this:
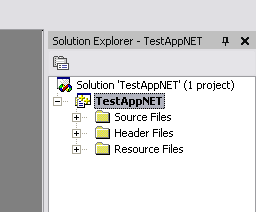
Now right click on the project (called TestAppNET here)
and click Properties.
You will be presented with a new dialog with which the project can be
configured.
In the Configuration list at the top of the dialog select All
Configurations.
First click Linker under the Configuration
Properties folder on the left side of the dialog. In the sub
sections of Linker click Input. On the
right side you should now see Additional Dependancies
at the top. This is where you add the external libraries that need to
be linked to your program at compile time. Add opengl32.lib,
glu32.lib, glaux.lib glut32.lib
and freeImage.lib to the field
in front of anything else that is there. (This field may be blank which
is Ok)
Note:
glu32.lib and glaux.lib are not needed
and thus are optional. However both contain some very useful functions
such as: gluPerspective(), gluLookAt(), auxDIBImageLoad(),
auxSolidSphere(), auxWireDodecahedron(),
etc. Use glaux.lib with caution because it may or may not be
platform independant.
Your dialog should now look nearly the same as the one pictured below.
Click OK.
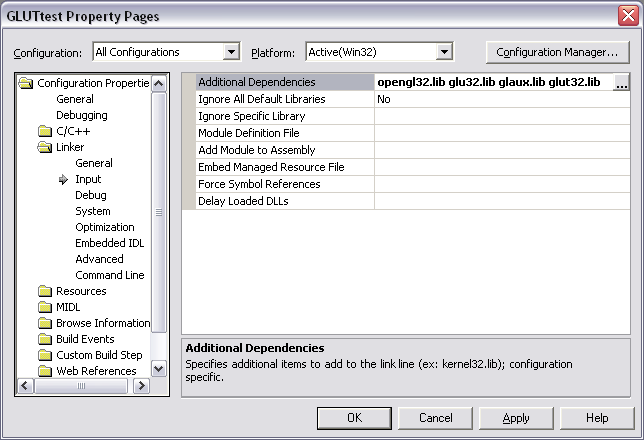
|
( 5 ) Turning off Precompiled Headers
|
Precompiled Headers play havoc with OpenGL and other
libraries such as STL. When you try to compile a number of strange
errors occur duing the linking phase. Turning off precompiled headers
solved this problem.
Right click on the project in the Solution Explorer and click
Properties , just as we did in the last
step. Select All Configurations at the top of the
dialog. Now click C/C++ on the left side. In the sub
sections of C/C++ click Precompiled Headers.
On the right side you should now see Create/Use Precompiled
Header, click it. A down arrow will appear, select Not
Using Precompiled Headers from the list. Click OK.
You may have to perform this action after you create the project. It
does not appear during the original project configuration.
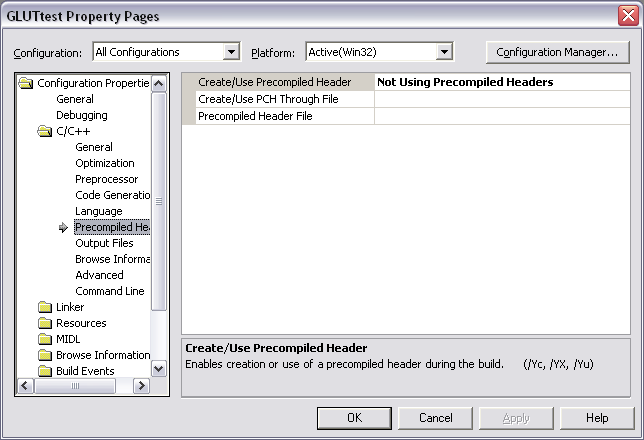
|
( 6 ) Creating a basic OpenGL/GLUT program
|
We now need a source code (.c or .cpp) file to put our
code into. To do this right click on the Source Files
folder under project in the Solution Explorer. If this is not
visible click the small + to the left of the project name. In the right
click menu click Add -> Add New Item.... A new
dialog will open. Click Visual C++ on the left side
then click C++ File (.cpp) on the right side.
You must give the new file a name in the Name field below.
Usually the default location is fine so leave it unchanged. (It
automatically puts it in the project directory)
Note:
The default filename ends with .cpp, but you can change this to .c by
specifying the complete name including the .c such as myCfile.c.
If you just enter myCfile it will add myCfile.cpp
to the project.
Once you have entered a name for the file click the Open
button.
The dialog will close and you should now have a blank file in the left
side of Visual Studio. We now need some code to get us going so cut and
paste the following into the empty file.
#include <windows.h> #include <GL/gl.h> #include <GL/glut.h>
int a[3]={10,10,10}, b[3]={10,-10,10}, c[3]={-10,-10,10}, d[3]={-10,10,10}, e[3]={10,10,-10}, f[3]={10,-10,-10}, g[3]={-10,-10,-10}, h[3]={-10,10,-10};
float angle=1.0;
void drawcube(void) { glClear(GL_COLOR_BUFFER_BIT); glColor3f(1.0, 1.0, 1.0);
glMatrixMode(GL_MODELVIEW); glRotatef(angle, 0.0, 1.0, 0.0); glBegin(GL_LINE_LOOP); glVertex3iv(a); glVertex3iv(b); glVertex3iv(c); glVertex3iv(d); glEnd(); glBegin(GL_LINE_LOOP); glVertex3iv(a); glVertex3iv(e); glVertex3iv(f); glVertex3iv(b); glEnd(); glBegin(GL_LINE_LOOP); glVertex3iv(d); glVertex3iv(h); glVertex3iv(g); glVertex3iv(c); glEnd(); glBegin(GL_LINE_LOOP); glVertex3iv(e); glVertex3iv(f); glVertex3iv(g); glVertex3iv(h); glEnd();
glFlush(); glutSwapBuffers(); }
void keyboard(unsigned char key, int x, int y) { switch (key) { case 0x1B: case 'q': case 'Q': exit(0); break; } }
void mouse(int btn, int state, int x, int y) { if (state == GLUT_DOWN) { if (btn == GLUT_LEFT_BUTTON) angle = angle + 1.0f; else if (btn == GLUT_RIGHT_BUTTON) angle = angle - 1.0f; else angle = 0.0f; } }
int main(int argc, char **argv) { glutInit(&argc, argv); glutInitWindowSize(500, 500); glutInitDisplayMode(GLUT_RGB | GLUT_DOUBLE); glutCreateWindow("Glut rotate"); glutMouseFunc(mouse); glutKeyboardFunc(keyboard); glutDisplayFunc(drawcube); glutIdleFunc(drawcube);
glMatrixMode(GL_PROJECTION); glLoadIdentity(); glOrtho(-30.0, 30.0, -30.0, 30.0, -30.0, 30.0); glRotatef(30.0, 1.0, 0.0, 0.0); glMatrixMode(GL_MODELVIEW); glClearColor(0.0, 0.0, 0.0, 0.0);
glutMainLoop(); return(0); }
Save the file now by clicking File -> Save filename
or by pressing Ctrl + S. Then run it by clicking Debug
-> Run without Debugging or by pressing Ctrl + F5.
Visual Studio should ask you if you want to compile the project so
click Yes. An OpenGL/GLUT window should now appear
with a wireframe box rotating. Press the Q key to
quit the program.
You are now ready to create your own openGL application,
congradulations.
:o)
|
(7 ) Creating a freeImage program.
|
We now need a second source code (.c or .cpp) file to
put our FreeImage code into. To do this right click on the Source
Files folder under project in the Solution Explorer.
If this is not visible click the small + to the left of the project
name. In the right click menu click Add -> Add New Item....
A new dialogue will open. Click Visual C++ on the
left side then click C++ File (.cpp) on the right
side.
Give the new file a name LoadFromHandle.cpp
in the Name field below. The default location is fine so
leave it unchanged. (It automatically puts it in the same project
directory as the openGL example)
Copy the following code into the new file. This
is a slightly modified version of the "LoadFromHandle.cpp" program
supplied with free image. The program should open a text window stating
the version etc. If it can load in a graphics file, then you should see
the following.
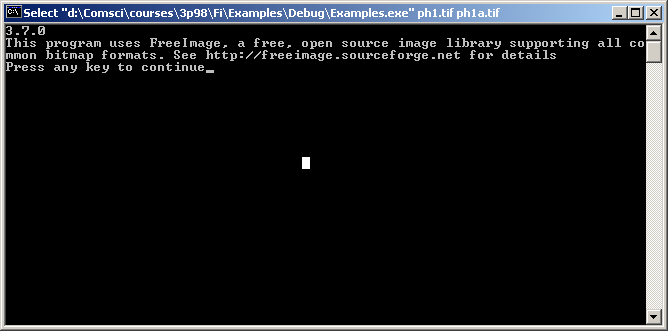
You will need
to specify the input file on the command line. Open the project
properties window and select debugging. Under Command Arguments
list any command line arguments your program is to accept. These are
read and made available to the program via the *argv[] strings. See below.
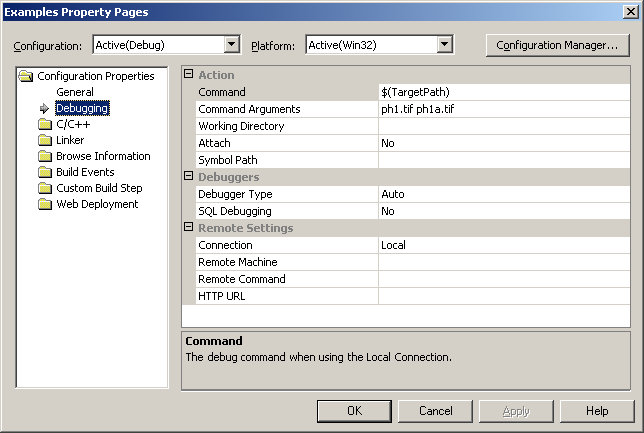
Here's an image used by the example: spit06.tif
#include "FreeImage.h"
typedef struct {
int r, g, b;
} pixel;
pixel **read_img(char *name, int *width, int *height) {
FIBITMAP *image;
int i,j;
RGBQUAD aPixel;
pixel **data;
if((image = FreeImage_Load(FIF_TIFF, name, 0)) == NULL) {
perror("FreeImage_Load");
return NULL;
}
*width = FreeImage_GetWidth(image);
*height = FreeImage_GetHeight(image);
data = (pixel **)malloc((*height)*sizeof(pixel *));
for(i = 0 ; i < (*height) ; i++) {
data[i] = (pixel *)malloc((*width)*sizeof(pixel));
for(j = 0 ; j < (*width) ; j++) {
FreeImage_GetPixelColor(image, j, i, &aPixel);
data[i][j].r = (aPixel.rgbRed);
data[i][j].g = (aPixel.rgbGreen);
data[i][j].b = (aPixel.rgbBlue);
}
}
FreeImage_Unload(image);
return data;
}
void write_img(char *name, pixel **data, int width, int height) {
FIBITMAP *image;
RGBQUAD aPixel;
int i,j;
image = FreeImage_Allocate(width, height, 24, 0, 0, 0);
if(!image) {
perror("FreeImage_Allocate");
return;
}
for(i = 0 ; i < height ; i++) {
for(j = 0 ; j < width ; j++) {
aPixel.rgbRed = data[i][j].r;
aPixel.rgbGreen = data[i][j].g;
aPixel.rgbBlue = data[i][j].b;
FreeImage_SetPixelColor(image, j, i, &aPixel);
}
}
if(!FreeImage_Save(FIF_TIFF, image, name, 0)) {
perror("FreeImage_Save");
}
FreeImage_Unload(image);
}
int main() {
pixel **data;
int w, h;
data = read_img("spit06.tif", &w, &h);
write_img("backup.tif", data, w, h);
return 0;
}
|
(8 ) Course Examples
|
There are 2 releases with working examples for
GLUT/FreeImage for Visual Studio (VS) and Linux. The same code has been
tested on each. Provided that VS and the Linux env. has been correctly
installed.
For Windows:
Download Win32Examples.zip, This file contains a VS
project by the same name. Extract the contents of this file to an
appropriate directory. Start VS and open the project file.
To build a specific example:
1). Select the .c file, right click and open the properties
for that file.
2). Excluded from build -> set to NO.
All other examples should be set to YES, VS will only
create one executable at a time using the given configuration. Note:
you can select all .c files, set them all to "Exclude from build", and
then include the one you are interested in.
Follow the instructions given in the tutorial for
compilation and execution.
Be sure to set appropriate command line arguments.
The file Examples.zip contains specific examples for
FreeImage. Only 1 example has been modified from the example suit
provided by FreeImage, that program is called "Example_read_mode_write.c".
This file should be compiled and executed. It gives a good example on
how to set up your own memory allocation, read an image into that
memory, modify the image and write it back out. It requires 2 command
line parameters. We have provided an input image example, ph1.tif
(Paris Hilton who else). Ensure you set the command line arguments to
include ph1.tif as the first parameter, the second can be anything you
want, include a valid suffix. The suffix determines the o/p file type.
Problems have been encountered running the example
without debugging. It tends to fail when allocating from the Heap. If
it fails to run, just use start (F5). The error should go away.
For Linux:
The same zip file may be extracted under Linux.
Preprocessor statements have been added to ensure that the proper
include files and function calls are appropriate for Linux.
To build the examples:
One make file has been created to build all executables
for the provided examples.
1). From a terminal window
type make. Provided the installation matches that which the
'make' file was created for, you should see each example being compiled
and linked. Should your installation be different, the you will need to
edit the 'Makefile' to include the proper paths for your installation.
2). Execute the
example, ---- ./NameOfExe
The Free image zip file can be extracted under Linux as
well. It has its own make file specific to building the "Example_read_mode_write.c"
example. Once again, the make file was created to reflect the lab
installation at Brock.
|
|