You should now be facing a blank project. Somewhere within Visual Studio should be
the Solution Explorer (It is docked on the right side at the top by default).
Note:
A Solution is the same as a Workspace if you are familar with the
Visual Studio 6.0/5.0 terminology.
If this windows does not exist click View -> Solution Explorer or
press Ctrl + Alt + L.
It will look similar to this:
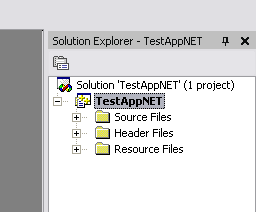
Now right click on the project (called TestAppNET here) and click Properties.
You will be presented with a new dialog with which the project can be configured.
In the Configuration list at the top of the dialog select All Configurations.
First click Linker under the Configuration Properties folder on the
left side of the dialog. In the sub sections of Linker click Input.
On the right side you should now see Additional Dependancies at the top.
This is where you add the external libraries that need to be linked to your program at compile
time. Add opengl32.lib, glu32.lib, glaux.lib
and glut32.lib to the field in front of anything else that is there.
(This field may be blank which is Ok)
Note:
glu32.lib and glaux.lib are not needed and thus are optional. However both
contain some very useful functions such as: gluPerspective(), gluLookAt(),
auxDIBImageLoad(), auxSolidSphere(), auxWireDodecahedron(), etc.
Use glaux.lib with caution because it may or may not be platform independant.
Your dialog should now look nearly the same as the one pictured below. Click OK.
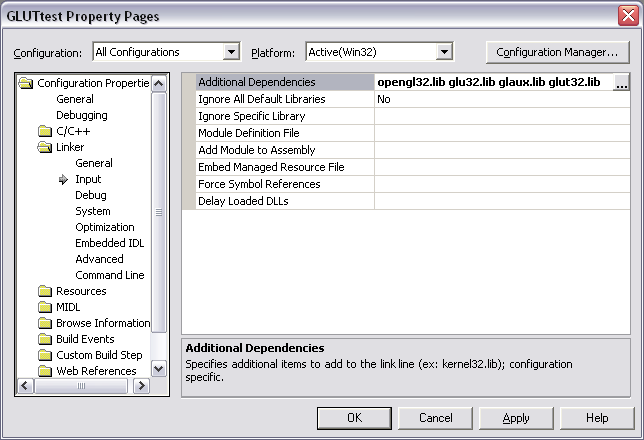
|
We now need a source code (.c or .cpp) file to put our code into. To do this right click on the
Source Files folder under project in the Solution Explorer. If this is
not visible click the small + to the left of the project name. In the right click menu click
Add -> Add New Item.... A new dialog will open. Click Visual C++
on the left side then click C++ File (.cpp) on the right side.
You must give the new file a name in the Name field below. Usually the default location
is fine so leave it unchanged. (It automatically puts it in the project directory)
Note:
The default filename ends with .cpp, but you can change this to .c by specifying the complete
name including the .c such as myCfile.c. If you just enter myCfile it will add
myCfile.cpp to the project.
Once you have entered a name for the file click the Open button.
The dialog will close and you should now have a blank file in the left side of Visual Studio.
We now need some code to get us going so cut and paste the following into the empty file.
#include <windows.h>
#include <GL/gl.h>
#include <GL/glut.h>
int a[3]={10,10,10}, b[3]={10,-10,10},
c[3]={-10,-10,10}, d[3]={-10,10,10},
e[3]={10,10,-10}, f[3]={10,-10,-10},
g[3]={-10,-10,-10}, h[3]={-10,10,-10};
float angle=1.0;
void drawcube(void)
{
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1.0, 1.0, 1.0);
glMatrixMode(GL_MODELVIEW);
glRotatef(angle, 0.0, 1.0, 0.0);
glBegin(GL_LINE_LOOP);
glVertex3iv(a);
glVertex3iv(b);
glVertex3iv(c);
glVertex3iv(d);
glEnd();
glBegin(GL_LINE_LOOP);
glVertex3iv(a);
glVertex3iv(e);
glVertex3iv(f);
glVertex3iv(b);
glEnd();
glBegin(GL_LINE_LOOP);
glVertex3iv(d);
glVertex3iv(h);
glVertex3iv(g);
glVertex3iv(c);
glEnd();
glBegin(GL_LINE_LOOP);
glVertex3iv(e);
glVertex3iv(f);
glVertex3iv(g);
glVertex3iv(h);
glEnd();
glFlush();
glutSwapBuffers();
}
void keyboard(unsigned char key, int x, int y)
{
switch (key)
{
case 0x1B:
case 'q':
case 'Q':
exit(0);
break;
}
}
void mouse(int btn, int state, int x, int y)
{
if (state == GLUT_DOWN)
{
if (btn == GLUT_LEFT_BUTTON)
angle = angle + 1.0f;
else if (btn == GLUT_RIGHT_BUTTON)
angle = angle - 1.0f;
else
angle = 0.0f;
}
}
int main(int argc, char **argv)
{
glutInit(&argc, argv);
glutInitWindowSize(500, 500);
glutInitDisplayMode(GLUT_RGB | GLUT_DOUBLE);
glutCreateWindow("Glut rotate");
glutMouseFunc(mouse);
glutKeyboardFunc(keyboard);
glutDisplayFunc(drawcube);
glutIdleFunc(drawcube);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(-30.0, 30.0, -30.0, 30.0, -30.0, 30.0);
glRotatef(30.0, 1.0, 0.0, 0.0);
glMatrixMode(GL_MODELVIEW);
glClearColor(0.0, 0.0, 0.0, 0.0);
glutMainLoop();
return(0);
}
Save the file now by clicking File -> Save filename or by
pressing Ctrl + S. Then run it by clicking Debug -> Run
without Debugging or by pressing Ctrl + F5. Visual Studio
should ask you if you want to compile the project so click Yes.
An OpenGL/GLUT window should now appear with a wireframe box rotating. Press the
Q key to quit the program.
You are now ready to create your own application, congradulations.
:o)
|